PlatformMaker
PlatformMaker is a platform puzzle game in which you can pick up blocks and place them to create your own platforms. The game features 35 levels and a level builder (more on that later). It also contains five types of blocks, each with its own functions. The green block standard, while the blue block falls until it hits something; the gray block is an on/off switch block type, and the purple block vanishes when you steps off it. A white block falls forever. With these blocks, you can make tons of different level ideas.
Project Info:
Solo Project
Project Time: until 14-02-2021
Engine: Unity
Code Languages: C#
Design Patterns: Singleton & FlyWeight
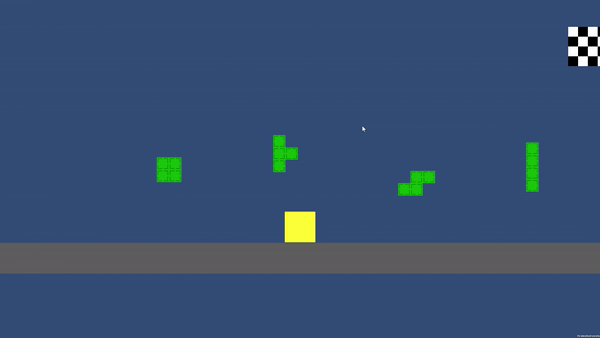
void Update()
{
if (!placed) //Checks if it's not placed
{
AllowPlacingCheck(); //Checks if it's allowed to be placed
Vector3 mousePos = Camera.main.ScreenToWorldPoint(Input.mousePosition);
mousePos.z = 0;
transform.position = mousePos;
if (Input.GetMouseButtonDown(0) && allowPlacing) //Places the block when it's allowed and the left mousebutton is down
{
placed = true;
gameObject.layer = 8;
sr.color = new Color32(255, 255, 255, 255);
if (type == blocktype.fall) rb.bodyType = RigidbodyType2D.Dynamic;
for (int i = 0; i < colliders.Length; i++)
{
colliders[i].isTrigger = false;
}
PlayerController.instance.somethingInHand = false;
} else if (Input.GetMouseButtonDown(1)) //Rotate when right mousebutton is pressed.
{
transform.Rotate(new Vector3(0, 0, 90));
}
}
if (placed && type == blocktype.falling)
{
transform.position = new Vector2(transform.position.x, transform.position.y - .4f * Time.fixedDeltaTime);
}
}
Place Function
LevelBuilder
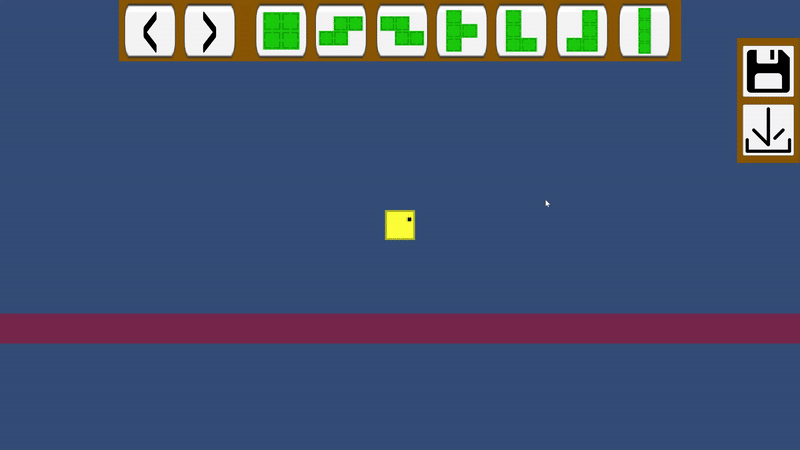
The Level Builder gives you access to all the blocks available in the regular levels. The game stores the blocks in a list, allowing you to save them at any time. When you load a level in the Level Builder, the blocks are placed both in the level and in the list. The Level Builder offers several options: you can move blocks, rotate them, turn them into pickups, delete them, and adjust their on/off state (if the block allows it). These functions are also keybound for easier control.
public void SaveAllData()
{
if (allPlaceableItems.Count > 0)
{
List blockSaveData = new List();
foreach (GameObject obj in allPlaceableItems)
{
LB_BlockPlaceData objBlockData = obj.GetComponent();
BlockSaveData tempData = new BlockSaveData //Creates BlockSaveData for everyBlock
{
position = obj.transform.position,
rotation = objBlockData.rotation,
pickUp = objBlockData.Pickup,
type = objBlockData.data.type.ToString(),
blockNumber = objBlockData.data.blockNumber,
onState = objBlockData.onState
};
blockSaveData.Add(tempData);
}
LB_SaveSystem.Save(blockSaveData, LB_SaveMenu.instance.customFileName + ".json"); //Send all the blockSaveData to SaveSystem.
}
}
public static void Save(List saveData, string _fileName)
{
string allJsonText = "";
string fileName = _fileName;
foreach (BlockSaveData data in saveData)
{
string json = JsonUtility.ToJson(data);
allJsonText += json + "\n";
}
if (Directory.Exists(Application.dataPath + "/CustomLevels")) File.WriteAllText(Application.dataPath + "/CustomLevels/" + fileName, allJsonText);
else
{
Directory.CreateDirectory(Application.dataPath + "/CustomLevels"); //Creates CustomLevels Folder when there isn't one
Save(saveData, _fileName);
}
}