Chourgie (V4)
Chourgie was created in the first year as a bot to track homework and tests. It originated from my first bot, Double, and uses a lot of the same code, though it's already more advanced than the old bot. Later, I had to update Chourgie to Discord.js V12 and now to V14, which required a lot of rewriting. Unfortunately, Discord.js often undergoes significant changes, causing some commands to break. Currently, Chourgie has 23 public commands and 1 private command.
Chourgie now uses the slashcommand system discord provides. In the early days Chourgie used ; as prefix.
Chourgie has a lot of new features one of them is the NS API. Chourgie is able to get the disruption and depature information
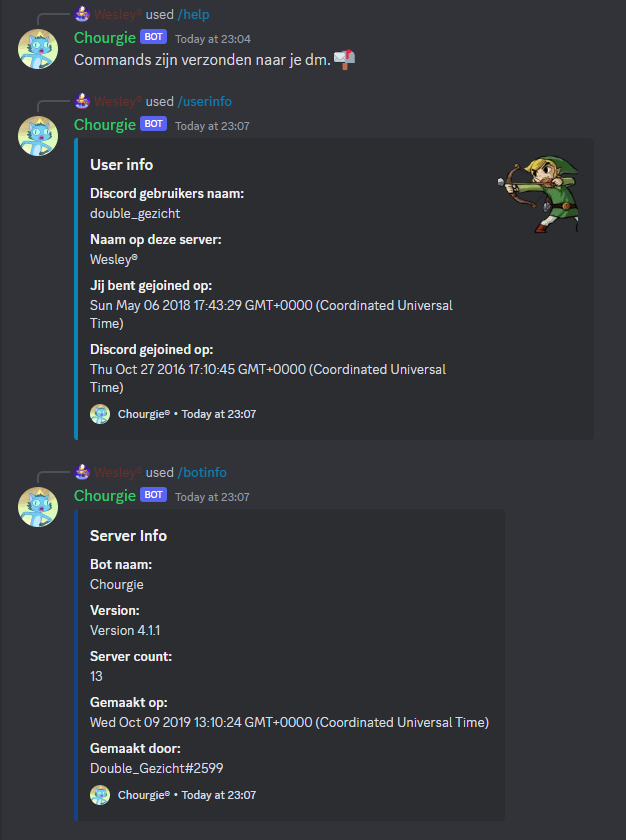
Project Info:
Solo Project
Project Timeline: First Year (MBO) until now
Code Languages: JavaScript, Discord.js
NS API
I use the NS API to display information to a user in an embed, utilizing Node-Fetch for fetching the API. The NS API doesn't always return content consistently, so some data fields, like "Extra reistijd," might be missing from parts of the response. I've filtered this out, and noticed that some data fields contain arrays of data.
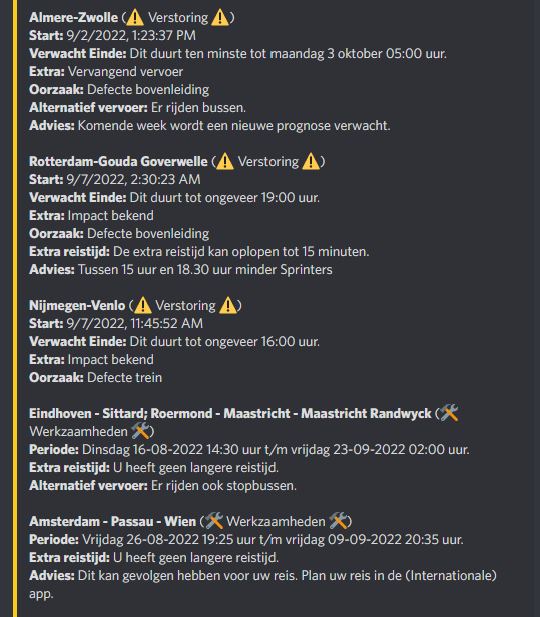
const discord = require("discord.js");
const fetch = require("node-fetch");
module.exports.run = async (client, message, args) => {
var botIcon = client.user.displayAvatarURL({ size: 2048 });
var apiKeyPrim = ":)";
fetch(`https://gateway.apiportal.ns.nl/reisinformatie-api/api/v3/disruptions?isActive=true`, {
method: 'GET',
headers: { "Ocp-Apim-Subscription-Key": apiKeyPrim },
})
.then(response => response.json())
.then(data => {
var info = "";
for (let i = 0; i < data.length; i++) {
var type = data[i].type
if (type == "CALAMITY") {
info += `**${data[i].title}** (ℹ Info ℹ)
**Bescrhijving:** ${data[i].description}\n`
}
else if (type == "DISRUPTION") {
info += `**${data[i].title}** (⚠ Verstoring ⚠)
**Start:** ${new Date(data[i].start).toLocaleString()}
**Verwacht Einde:** ${data[i].expectedDuration.description}
**Extra:** ${data[i].phase.label}\n`
let timeSpans = "";
for (let t = 0; t < data[i].timespans.length; t++) {
timeSpans += `**Oorzaak:** ${data[i].timespans[t].cause.label}\n`
if (data[i].timespans[t].alternativeTransport) timeSpans += `**Alternatief vervoer:** ${data[i].timespans[t].alternativeTransport.label}\n`
if (data[i].timespans[t].additionalTravelTime) timeSpans += `**Alternatief duur vervoer:** ${data[i].timespans[t].additionalTravelTime.label}\n`
if (data[i].timespans[t].advices && data[i].timespans[t].advices.length != 0) {
timeSpans += "**Advies:** "
for (let a = 0; a < data[i].timespans[t].advices.length; a++) {
timeSpans += data[i].timespans[t].advices[a] + "\n";
}
}
timeSpans += "\n"
}
info += timeSpans
} else if (type == "MAINTENANCE") {
info += `**${data[i].title}** (🛠 Werkzaamheden 🛠)
**Periode:** ${data[i].period}
**Extra reistijd:** ${data[i].summaryAdditionalTravelTime.label}\n`
let timeSpans = "";
for (let t = 0; t < data[i].timespans.length; t++) {
if (data[i].timespans[t].alternativeTransport) timeSpans += `**Alternatief vervoer:** ${data[i].timespans[t].alternativeTransport.label}\n`
if (data[i].timespans[t].advices && data[i].timespans[t].advices.length != 0) {
timeSpans += "**Advies:** "
for (let a = 0; a < data[i].timespans[t].advices.length; a++) {
timeSpans += data[i].timespans[t].advices[a] + "\n";
}
}
timeSpans += "\n"
}
info += timeSpans
}
}
var nsEmbed = new discord.MessageEmbed()
.setColor("#fcc81c")
.setTitle("Nederlandse Spoorwegen Verstoringen")
.setDescription(info)
.setFooter("Chourgie®", botIcon)
.setTimestamp();
return message.channel.send({ embeds: [nsEmbed] })
}).catch(err => console.log(err));
}
module.exports.help = {
name: "ns",
category: "general",
description: "NS informatie."
}
NS API Code for disruptions.