Econeer
During Project Context II, we worked on a game commissioned by ROM Utrecht. The assignment was to develop a game based on an ecosystem. Econeer is a survival game with a dynamic ecosystem, in which the player takes on the role of a biologist exploring a mysterious island. The goal is to discover the island's unique animals and survive without drastically disrupting the ecosystem. The island reacts to your actions… Gather data, track animal populations, and try to survive in harmony with nature!
Project Info:
Team members: Jim Dekker, Mitchel Gereadts, Julius Kamphuis, Joey van Gool, Griffin Linckens & Emma Bout
Project duration: HKU Year 2, Period 3 (10-02-2025 until 28-03-2025)
Engine: Unity
Code Languages: C#
Design Patterns: Observer, Singleton & FlyWeight
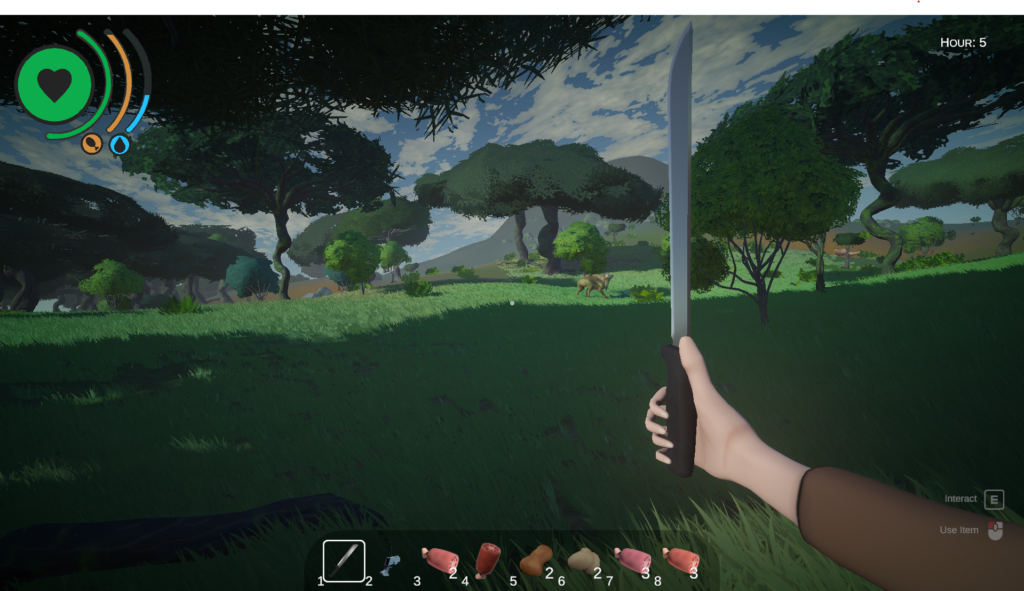
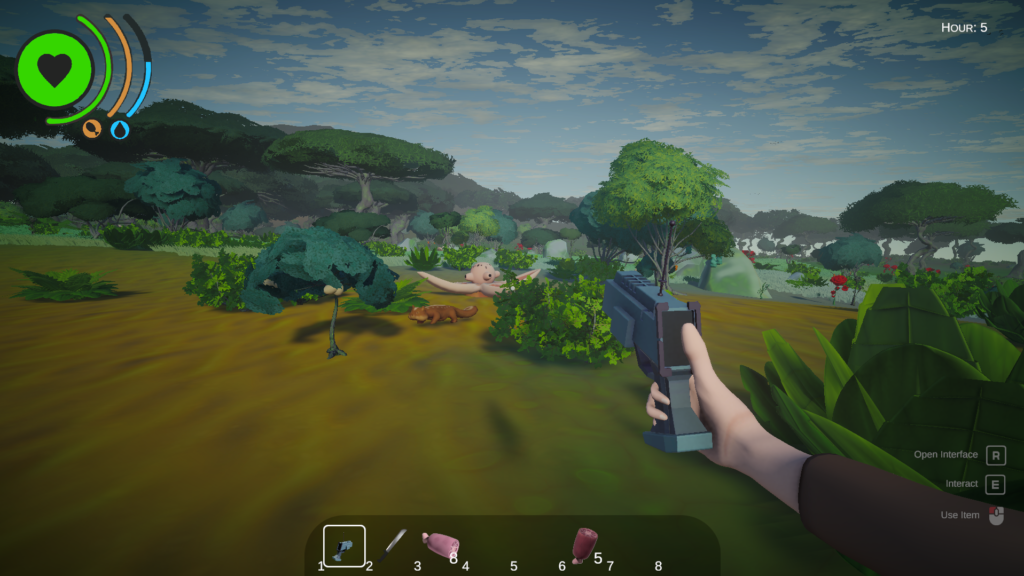
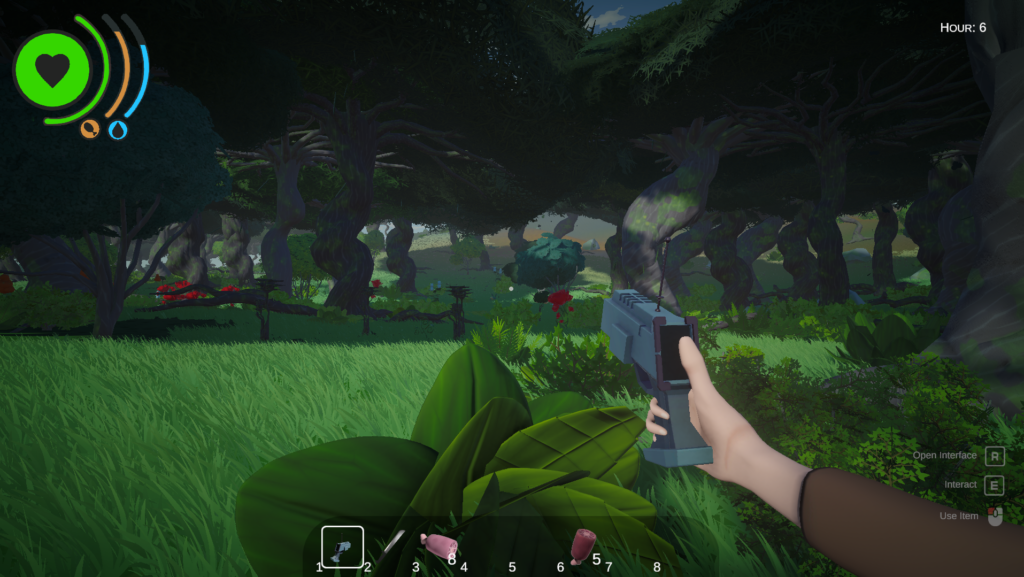
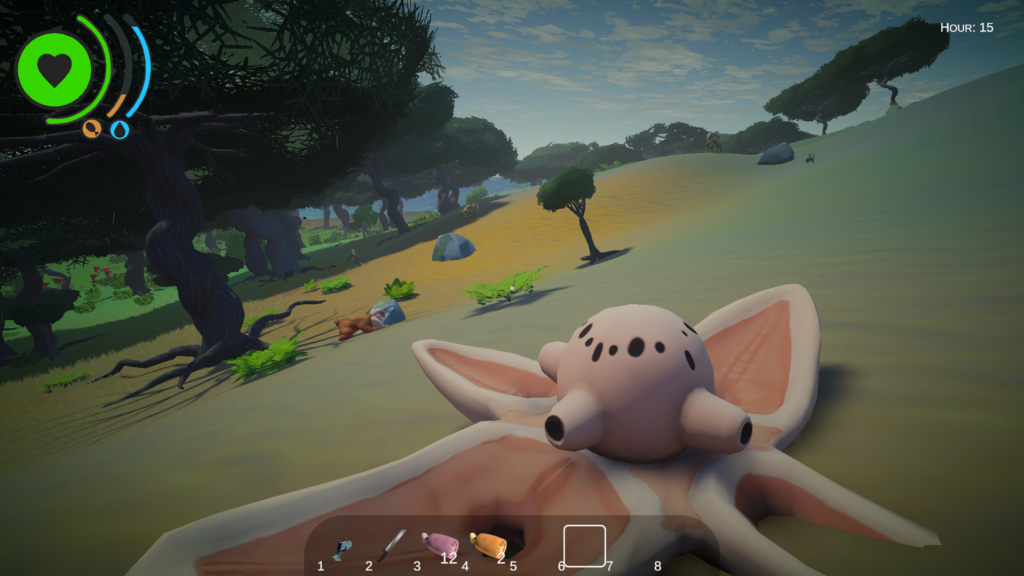
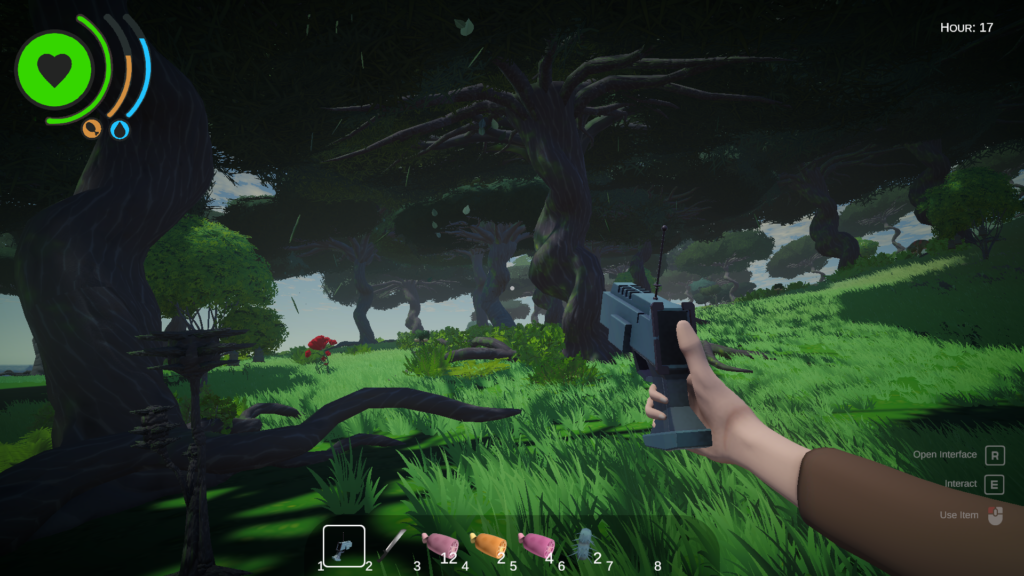
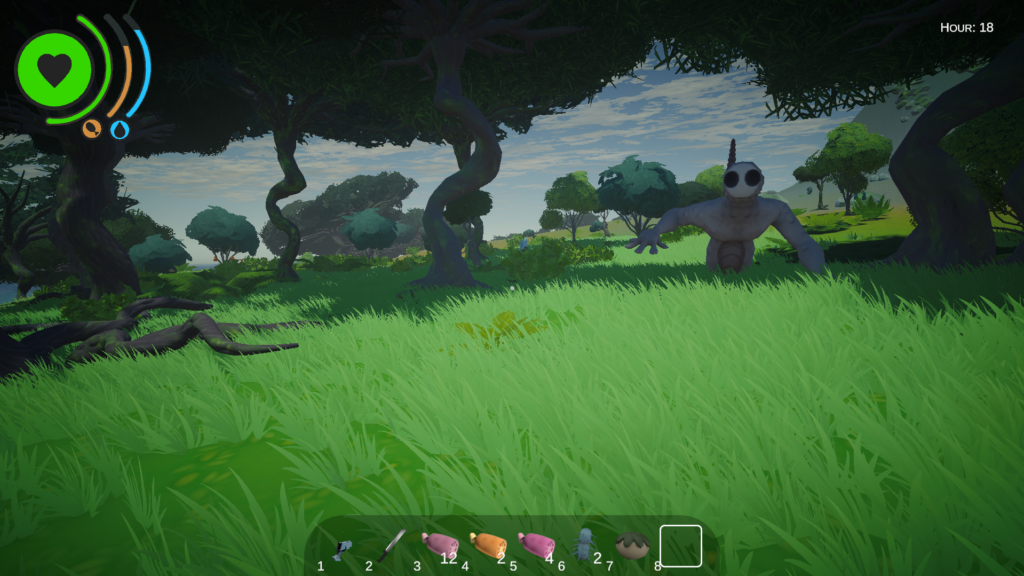
Animal GOAP (Goal-Oriented Action Planning)
GOAP (Goal-Oriented Action Planning) is an AI system in which an agent is given a goal and independently determines which actions are needed to achieve that goal. Instead of following fixed behavior rules, GOAP dynamically generates a plan by analyzing available actions and how they connect. The AI starts with the end goal and works backward to form a sequence of actions that align with each other. Each action has a precondition that must be met (which can be fulfilled by a previous action). The planner searches for the most efficient route while considering the current situation. GOAP is highly flexible compared to traditional AI, as the agent can adapt to changing circumstances without requiring manually scripted scenarios.
public Queue<GAction> Plan(List<GAction> actions, Dictionary<string, int> goal, WorldStates beliefstates)
{
List<GAction> useableActions = new List<GAction>();
foreach (GAction a in actions)
{
if (a.IsAchievable()) useableActions.Add(a);
}
List<Node> leaves = new List<Node>();
Node start = new Node(null, 0, GWorld.Instance.GetWorld().GetStates(), beliefstates.GetStates(), null);
bool succes = BuildGraph(start, leaves, useableActions, goal);
if (!succes) return null;
Node cheapest = null;
foreach (Node leaf in leaves)
{
if (cheapest == null) cheapest = leaf;
else
{
if (leaf.cost < cheapest.cost) cheapest = leaf;
}
}
List<GAction> result = new List<GAction>();
Node n = cheapest;
while (n != null)
{
if (n.action != null)
{
result.Insert(0, n.action);
}
n = n.parent;
}
Queue<GAction> queue = new Queue<GAction>();
result.ForEach(a => queue.Enqueue(a));
return queue;
}
Creates a plan with all the actions assigned to the animal, attempting to make it as cost-effective as possible.
Animal Script
The Animals script is the base class for all animals. This class manages functions such as: growing babies, food reduction, and handling pregnancies. The animals themselves have an additional GOAP layer on top. In their specific animal-type script, different animals' goals are defined. Goals include: Wander, Fed, FoundMate, HasBred, and IsSafe. Dynamic goals are also added when necessary. For example, Panic is added when an animal takes damage.
private void HandleReproduction()
{
timeSinceLastMating = Mathf.Max(timeSinceLastMating - TimeTickSystem.ANIMAL_TICK_RATE, 0);
if (Sex == Sex.Male) EvaluateReproduction();
if (IsPregnant) UpdatePregnancy();
}
private void EvaluateReproduction()
{
beliefs.RemoveState("canReproduce");
if (IsBaby) return;
if (timeSinceLastMating > 0) return;
if (GetHungerPercentage() < 70) return;
if (inventory.FindItemWithKey("partner") != null) return;
if (currentAction != null && currentAction.GetType() == typeof(AFlee)) return;
beliefs.SetState("canReproduce", 1);
}
Managing reproduction. This is the male part where it receives the belief "canReproduce". Now, through its GOAP, it can make a request to a female.
Inventory System
The player has a hotbar and the option for a backpack. Currently, the backpack is disabled, meaning the player can only store items in the hotbar. However, the original system was designed to automatically place items in the backpack when the hotbar is full. When the player interacts with an object in the world, the system can query which item the player is currently holding. This is determined by the contents of the hotbar. If the player performs an action that requires a specific item, the system will check if that item is present in the hotbar.
public bool AddToInventory(InventoryItemData itemToAdd, int amountToAdd)
{
//Checks wheter Item exist in inventory.
if (ContainsItem(itemToAdd, out List<InventorySlot> invSlot))
{
foreach (InventorySlot slot in invSlot)
{
if (slot.RoomLeftInStack(amountToAdd))
{
slot.AddToStack(amountToAdd);
OnInventorySlotChanged?.Invoke(slot);
return true;
}
}
}
//Gets the first available slot.
if (HasFreeSlot(out InventorySlot freeSlot))
{
freeSlot.UpdateInventorySlot(itemToAdd, amountToAdd);
OnInventorySlotChanged?.Invoke(freeSlot);
return true;
}
return false;
}
public bool ContainsItem(InventoryItemData itemToAdd, out List<InventorySlot> invSlot)
{
invSlot = inventorySlots.Where(i => i.ItemData == itemToAdd).ToList();
return invSlot != null;
}
public bool HasFreeSlot(out InventorySlot freeSlot)
{
freeSlot = inventorySlots.FirstOrDefault(i => i.ItemData == null);
return freeSlot != null;
}
Adding new items and checking available space.