HKU Game Blitz
For Project Context, we had to create a game for the HKU open day. The game needed to reflect the study experience at HKU. We chose to represent the three main programs and the time pressure that exists at HKU. The game was intended to be played by a prospective student and one of their parents. Since we all know that parents often struggle with controls, we decided to use the Wiimote as input. The Wii is a console designed for families, so it was a logical choice.
For the Art mini-game, we created a painting game where both players had to paint. In the Design mini-game, one player built a level while the other had to complete it. In the Development mini-game, both players had to write an instruction set to get a robot to the finish.
Project Info:
Team Members: Floris Koelemaij, Lloyd Belterman, Arman Aryob, ELL Visser
Project time: HKU Year 1 Period 3 (2024)
Engine: Unity
Code Languages: C#
Design Patterns: Singleton & FlyWeight
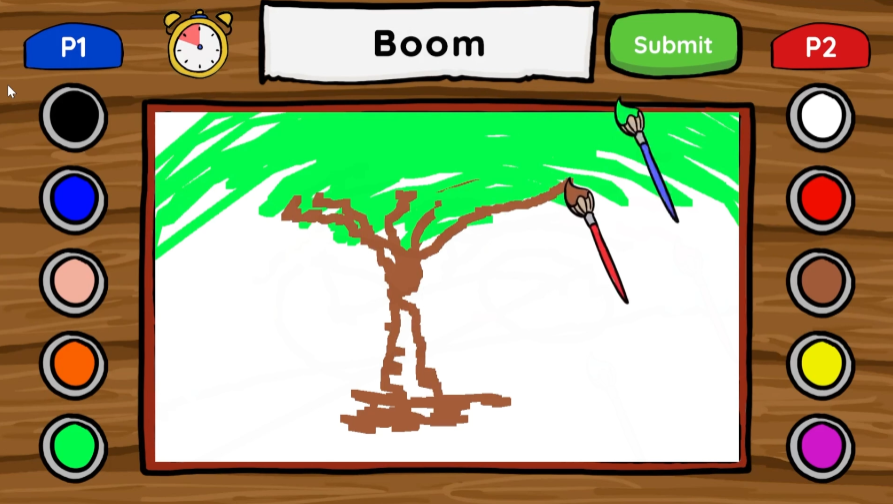
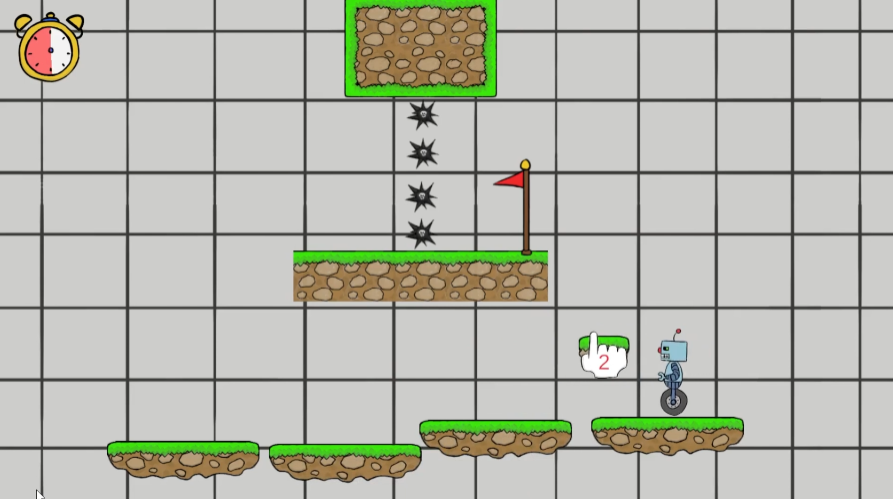
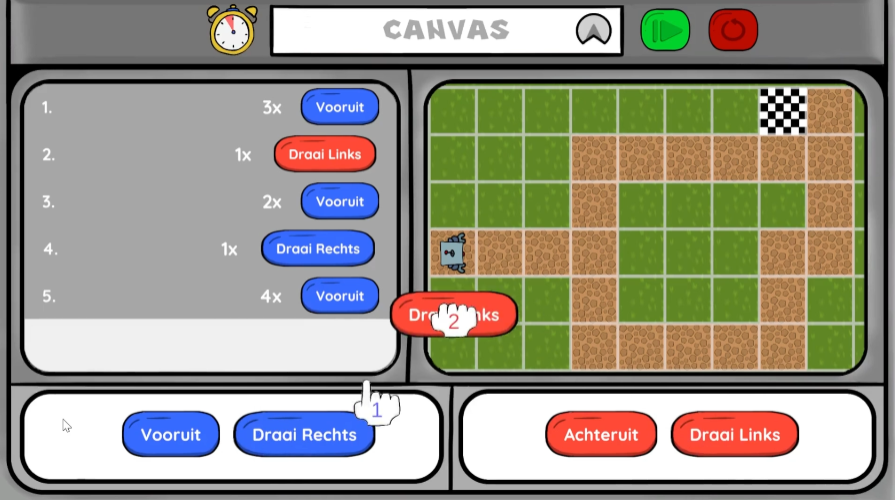
Wiimotes
The game uses Wiimotes, for which I used a WiiMote API (https://github.com/Flafla2/Unity-Wiimote). Unfortunately, I couldn't get the API to work for the newer Wiimotes (WiimotionPlus), so it only works for the older Wiimotes. The vibration of the pointers occurred because the Wiimotes provided very sensitive input. Smoothing the input (lerping) didn’t give the desired result and made the input feel laggy. I modified the WiiMote API and built a custom input handler, which allowed us to mimic a Wiimote using the mouse. This made testing easier, but the Wiimote API had a strange input handler.
protected void HandleInput()
{
foreach (KeyValuePair key in activeInput)
{
if (key.Value)
{
if (!buttonDown.ContainsKey(key.Key))
{
buttonDown.Add(key.Key, Time.frameCount + 1);
}
}
else if (buttonDown.ContainsKey(key.Key))
{
buttonDown.Remove(key.Key);
if (!buttonUp.ContainsKey(key.Key)) buttonUp.Add(key.Key, Time.frameCount + 1);
else buttonUp[key.Key] = Time.frameCount + 1;
}
}
}
Handles the Wiimote input.
Painting Game
In the painting game, the player receives a random prompt from the game, and both players must work together to draw it. Each player has a set of colors, and they cannot use each other’s colors. At the end, the drawing is reviewed. We wanted to implement an automatic review system, but it was too complex for the time available. The drawing takes place on a Texture2D. First, all the drawing data is collected, and then it’s applied to the Texture2D. (The system isn’t limited to just 2 players.)
public void DrawPixels(Dictionary|int, DrawData| wiiPositions)
{
Texture2D texture2D = currentDrawing;
RenderTexture curText = RenderTexture.active;
RenderTexture renTex = new RenderTexture(texture2D.width, texture2D.height, 32);
Graphics.Blit(texture2D, renTex);
RenderTexture.active = renTex;
texture2D.ReadPixels(new Rect(0, 0, texture2D.width, texture2D.height), 0, 0);
foreach (KeyValuePair pos in wiiPositions)
{
Vector2 newPos = pos.Value.position - new Vector2(Screen.width * .5f, Screen.height * .5f);
newPos += new Vector2(drawingImage.rectTransform.rect.width * drawCanvas.scaleFactor * .5f, drawingImage.rectTransform.rect.height * drawCanvas.scaleFactor * .5f);
newPos = new Vector2(newPos.x / (1238 * drawCanvas.scaleFactor) * width, (newPos.y + 75 * drawCanvas.scaleFactor) / (740 * drawCanvas.scaleFactor) * height);
Vector2Int changePixelPos = new Vector2Int(Mathf.RoundToInt(newPos.x), Mathf.RoundToInt(newPos.y));
List positions = lastDrawData.ContainsKey(pos.Key) ? GetLinearPositions(lastDrawData[pos.Key].position, changePixelPos) : new List() { changePixelPos };
foreach (Vector3 position in positions)
{
List pixels = GetNeighbouringPixels(new Vector2(texture2D.width, texture2D.height), position, brushRadius);
if (pixels.Count > 0)
foreach (Vector2 p in pixels)
texture2D.SetPixel((int)p.x, (int)p.y, pos.Value.color);
}
if (!lastDrawData.ContainsKey(pos.Key)) lastDrawData.Add(pos.Key, new DrawData(newPos, pos.Value.color));
else lastDrawData[pos.Key] = new DrawData(newPos, pos.Value.color);
}
texture2D.Apply();
RenderTexture.active = curText;
renTex.Release();
Destroy(renTex);
curText = null;
renTex = null;
drawingImage.texture = texture2D;
}
Draws all pixels on the texture2D
Programming Game
In the programming mini-game, the goal is to get the robot from point A to point B by using code blocks. The players have 4 simple code blocks: Move Forward, Move Backward, Turn Left, and Turn Right. Each player has 2 code blocks. After writing the instruction set, they can run it. Once the instructions are set, the players can’t add or change anything (evaluation phase). The robot follows the complete instruction set, which is shown on the screen. If an action is not possible, the robot will stop.
{ //(Display fix)
public void AddToCode(DevPlaceButton placeButton)
{
if (codeLines.Count > 0)
{
CodeLines lastIndex = codeLines[codeLines.Count - 1];
if (lastIndex.PlaceButtonName == placeButton.name)
{
lastIndex.AddRunCount();
Destroy(placeButton.gameObject);
return;
}
}
CodeLines tempLine = Instantiate(codeLinePrefab, codeLineParent);
codeLines.Add(tempLine);
tempLine.Setup(codeLines.Count, placeButton);
if (codeLines.Count > 6)
{
Vector2 viewPortLocalPosition = scrollRect.viewport.localPosition;
Vector2 targetLocalPosition = tempLine.transform.localPosition;
Vector2 newTargetPosition = new Vector2(codeLineParent.transform.localPosition.x, 0 - (viewPortLocalPosition.y + targetLocalPosition.y + scrollRect.viewport.rect.height * .38f));
codeLineParent.localPosition = newTargetPosition;
}
}
private IEnumerator RunCode()
{
int executionIndex = 0;
while (true)
{
if (codeLines[executionIndex].RunCode(out int ActionIndex))
{
executionIndex++;
}
robotPlayer.SendInstructions(ActionIndex);
yield return new WaitForSeconds(1);
if (codeLines.Count == executionIndex) break;
}
if (robotPlayer.IsOnFinish())
{
//FINISH
GameManager.Instance.AddScore(1);
GameManager.Instance.LoadNextGame();
}
else
{
GameManager.Instance.LoadNextGame();
}
}
}
dds a code block to the code execution and the Code run IEnumerator.
No download available as it needs to be played with Wiimotes.