Ivannio Ivre, The Painter’s Struggle
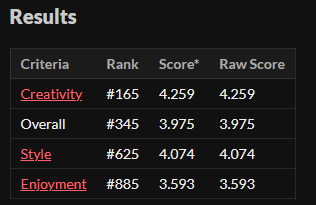
I participated in the GMTK 2024 game jam with a small team. The theme was "Built to Scale". After a long brainstorming session, we came up with a painting game in wich the size of the brush constantly changes size. We also thought of other scale-related modifiers, but they couldn't be implemented. The story is that Ivannio is a drunk painter, and he can't maintain a steady brush size. You need to draw six prompts based on customer requests. After drawing, you can choose where to place your painting in the gallery. Once you've created six drawings, you can upload your gallery to be rated by other players, and you can also rate other players' galleries.
The end result turned out really well, and we ended up in a great overall position that we didn't expect 345th out of 7.605 participants. That's a solid top 500 finish. Our goal was to at least be in the top 2.000, and we achieved that. Every category also ranked in the top 1.000.
Project Info:
Team Members: Floris Koelemaij, Andrich Laker, Tristan Laman
Project Time: 16-08-2024 until 20-08-2024
Engine: Unity
Code Languages: C#, PHP
Game Jam: GMTK 2024
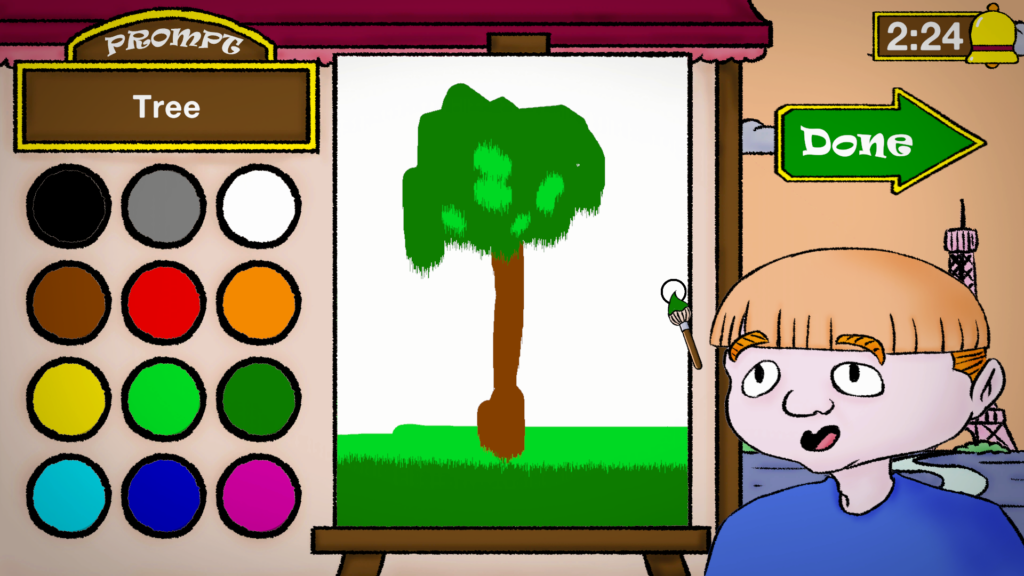
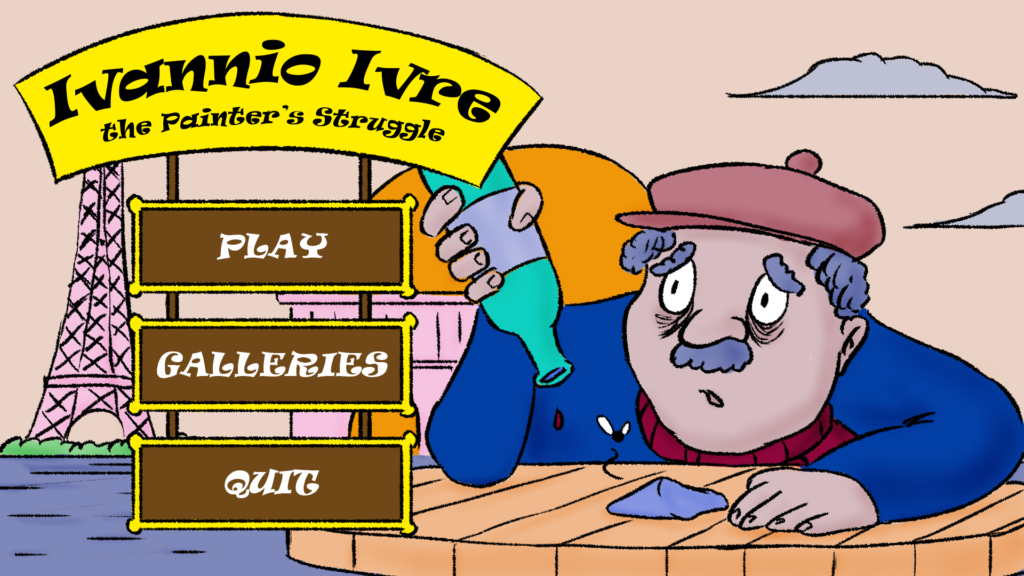
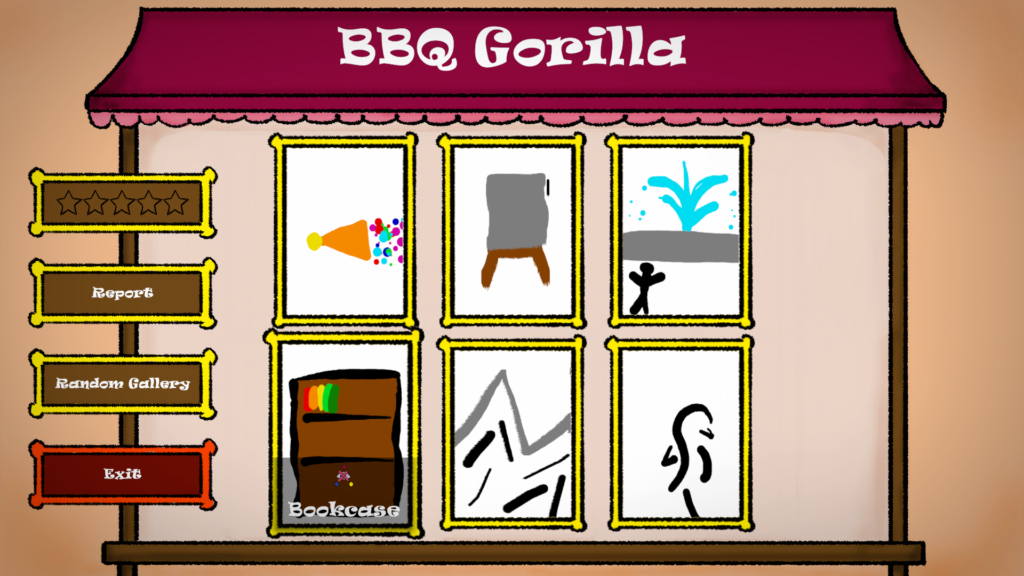
Painting
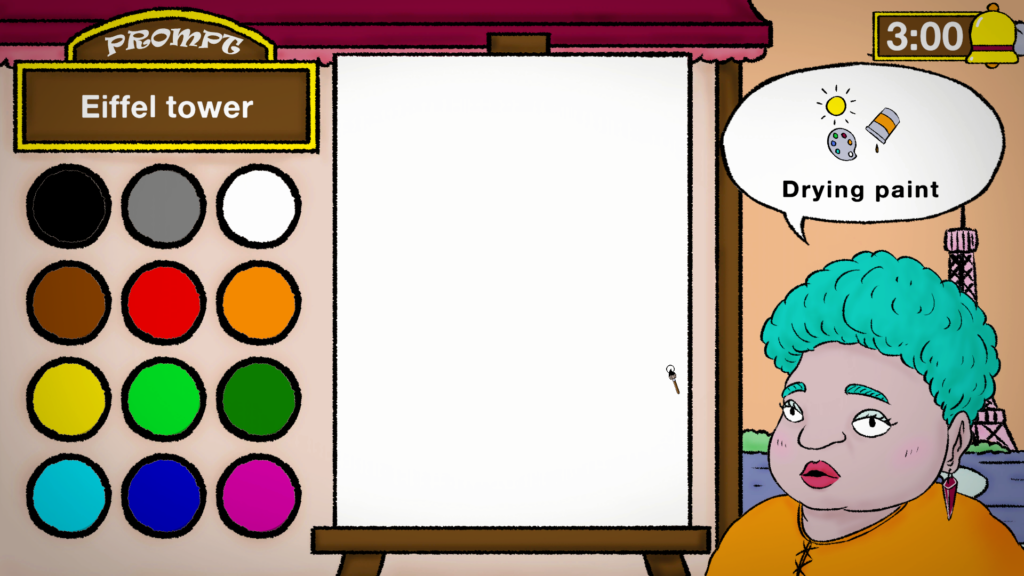
The player receives a random prompt from the customer that they have to draw. The drawing works on a Texture2D. Various modifiers can also affect the canvas, so all the draw data is first gathered, and then the modifier edits are processed. The wet paint and wind effects caused some performance issues since they had to update every pixel to achieve the desired effect. After some optimization, the game no longer lagged when the wet paint effect was applied. Once the player finished drawing, they could click "done" to go to the gallery and choose a spot for their painting.
private void WetPaint()
{
Color32[] pixels = currentDrawing.GetPixels32();
for (int y = 1; y < currentDrawing.height; y++)
{
for (int x = 0; x < currentDrawing.width; x++)
{
int index = y * currentDrawing.width + x;
Color32 newColor = pixels[index];
if(IsSameColor(newColor, backgroundColor)) continue;
if (!QuickRandom()) continue;
int aboveIndex = index - currentDrawing.width;
if (aboveIndex >= 0)
{
Color32 checkColor = pixels[aboveIndex];
if (IsSameColor(newColor, checkColor)) continue;
pixels[aboveIndex] = newColor;
}
}
}
currentDrawing.SetPixels32(pixels);
currentDrawing.Apply();
}
Wetpaint function. Goes through all the pixels and checks if there is a color. Yes? Is the random value also correct? Update the pixel below.
API
The game uses an API to upload paintings and view galleries. This API is hosted on the API version of this website (https://api.wesleydegraaf.com). I fully developed this API and can do the following: upload galleries (including images), list galleries, view a gallery, rate a gallery, report a gallery, and for the developer, there's a gallery report viewer.
require_once("GMTKconnection.php");
$galleryId = $_GET['id'] ?? null;
if ($galleryId) {
$sql = "SELECT GalleryName, Rating
FROM Gallery
WHERE ID = ?";
$stmt = $conn->prepare($sql);
$stmt->bind_param("i", $galleryId);
$stmt->execute();
$result = $stmt->get_result();
$response = new stdClass();
$response->gallery = null;
$response->status = "FAILED";
if ($result->num_rows > 0) {
$row = $result->fetch_assoc();
$gallery = new stdClass();
$gallery->galleryId = $galleryId;
$gallery->galleryName = $row["GalleryName"];
$gallery->rating = $row["Rating"];
$sqlPhotos = "SELECT ID, Name, ImgDir, Modifier
FROM Paintings
WHERE GalleryID = ?";
$stmtPhotos = $conn->prepare($sqlPhotos);
if ($stmtPhotos === false) {
die('Prepare failed: ' . $conn->error);
}
$stmtPhotos->bind_param("i", $galleryId);
$stmtPhotos->execute();
$photosResult = $stmtPhotos->get_result();
$gallery->photos = array();
while ($photo = $photosResult->fetch_assoc()) {
$photoObj = new stdClass();
$photoObj->paintingName = $photo["Name"];
$photoObj->imgDir = $photo["ImgDir"];
$photoObj->modifier = $photo['Modifier'];
$gallery->photos[] = $photoObj;
}
$response->gallery = $gallery;
$response->status = "OK";
$response->customMessage = "Gallery and photos retrieved successfully.";
} else {
$response->customMessage = "Gallery not found.";
}
$stmt->close();
$conn->close();
} else {
$response = new stdClass();
$response->status = "FAILED";
$response->customMessage = "No gallery ID provided.";
}
echo json_encode($response);
Get the gallery with the specified ID. Returns: GalleryID, GalleryName, Rating, and photos [photo name, img location, modifier].