Next Station
For project Vrij, we had to create a game based on an experience from our study trip to Berlin. During the trip, we visited a park around 9:35 PM, but when we arrived, we saw that it closed at 10:00 PM. We hesitated but decided to take a quick look and we would head back. There was only limited light in the park, and as we walked further, it got darker and darker. Our goal was to reach one of the old FLAK towers in Berlin. To do this, we had to leave the main path of the park and head into an unlit area. We walked around with a flashlight. In the shadows, strange shapes appeared, and at times, it seemed like the movement of an animal.
We transformed this experience, along with some other small experiences from Berlin, into Next Station. Next Station is an escape room-style game where the player must solve two puzzles to escape. However, there's something strange going on. When the player completes one loop, they’ve actually only completed half a loop. The player must walk two loops to finish one full loop. Additionally, when the player moves forward, time moves forward, and when they walk backward, time moves in reverse.
The player needs to meet their friend at South Station, but to do so, they must first buy a ticket. When the player purchases the ticket, all the power in the station goes out. The player must then find another way to get a ticket. Once the player obtains the ticket, they must ensure the train arrives at the correct time. When the train arrives at the station and the player has a ticket, they can board the train. The game ends with a humorous twist, making the player think the game is over, only to have the real ending occur just a bit later.
Project Info:
Team Members: Louiza Kevorkian, Isabella van den Dungen
Project time: HKU Year 1 Period 4
Engine: Unity
Code Languages: C#
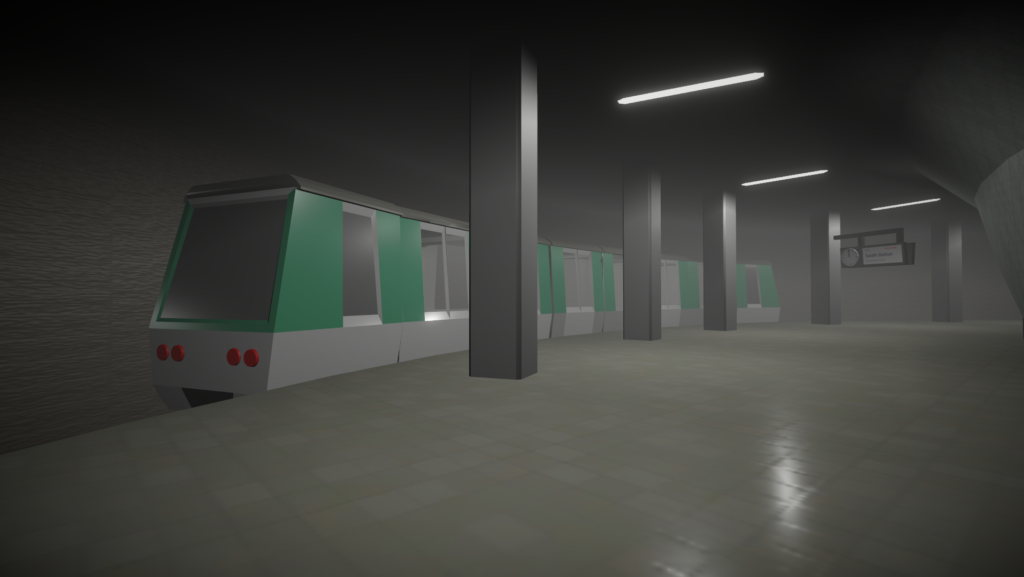
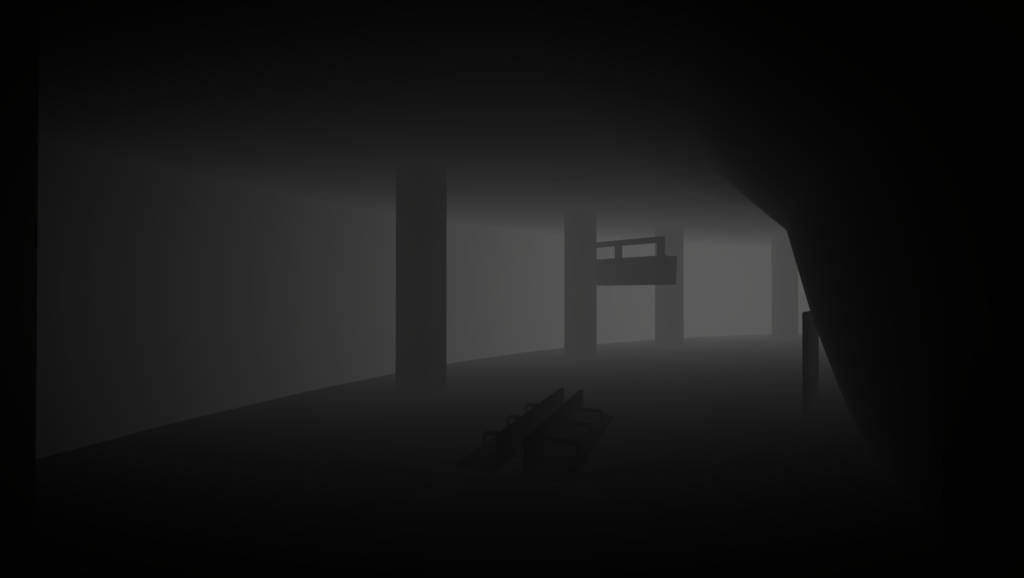
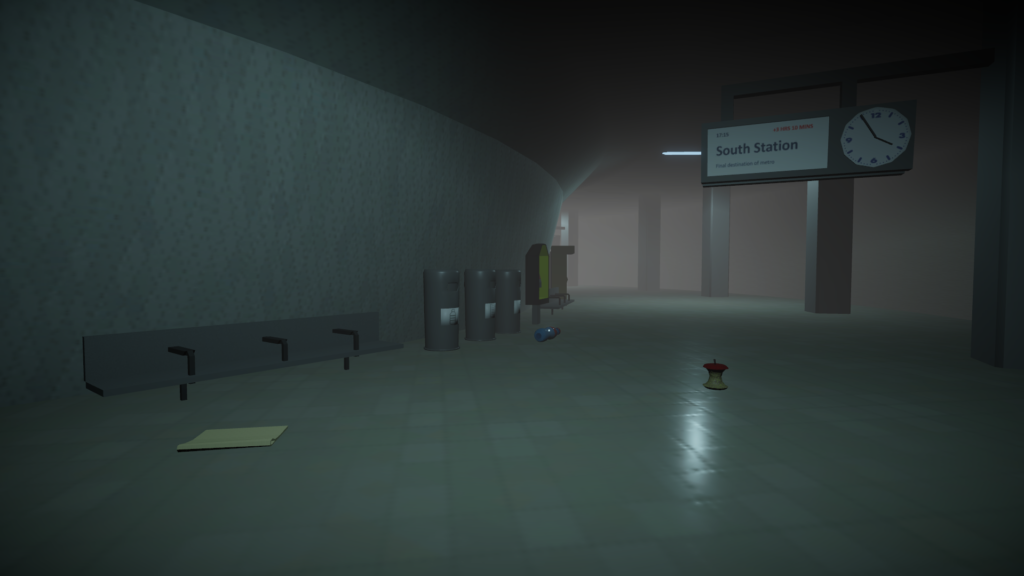
Looping Station System
The station is made up of 8 quarter segments. Each time the player crosses a segment, another section further along is loaded. The player needs to walk two loops to complete one full loop, which is meant to confuse them. The player can walk in either direction, and this also affects the arrival puzzle. In the arrival puzzle, the player must ensure that the time matches the time displayed on the board for the train to arrive.
public void NextPart(int checkpoint)
{
if ((lastCheckpoint > checkpoint && !(lastCheckpoint == 4 && checkpoint == 1)) || (lastCheckpoint == 1 && checkpoint == 4))
{
currentIndex--;
lastCheckpoint = checkpoint;
if (currentIndex < 0) currentIndex = mapSections.Count - 1;
OnNextPart?.Invoke(SectionDir.MIN);
}
else if (lastCheckpoint < checkpoint || (lastCheckpoint == 4 && checkpoint == 1))
{
currentIndex++;
lastCheckpoint = checkpoint;
if (currentIndex >= mapSections.Count) currentIndex = 0;
OnNextPart?.Invoke(SectionDir.PLUS);
}
OnSectionUpdate?.Invoke(currentIndex);
Load();
}
Show a new segment from the metro station
Ending
For the time puzzle, the train must arrive at the correct segment because the time increments are based on the direction the player walks, and for how long? However, we don’t know exactly where the train should arrive. After a small calculation, this wasn’t too difficult to implement. The only issue was that sometimes the metro’s animation would move in the wrong direction. For the departure, the metro needed to drive away, and once it reached the opposite side, it would despawn.
public void DriveAway()
{
int currentRotationIndex = SectionManager.Instance.GetCurrentCheckpoint() - 1;
transform.DORotate(new Vector3(0, currentRotationIndex * 90 - 45 + 179, 0), 6f, RotateMode.Fast).SetEase(Ease.InQuad).OnComplete(() =>
{
metroObject.SetActive(false);
blockDespawn = false;
});
}
private void DriveToPlatform()
{
int currentRotationIndex = SectionManager.Instance.GetCurrentCheckpoint() - 1;
transform.rotation = Quaternion.Euler(new Vector3(0, currentRotationIndex * 90 - 45 - 179, 0));
Sequence sequence = DOTween.Sequence();
sequence.Append(transform.DORotate(new Vector3(0, currentRotationIndex * 90 - 45, 0), 6f).SetEase(Ease.OutQuad))
.AppendInterval(2f)
.AppendCallback(() => OpenDoor(true))
.Play();
}
The departure and arrival from/at the station