Signaller
During an elective course, we had to create a game for a museum. We chose the Spoorwegmuseum. Signaller is a game where the player takes on the role of a train traffic controller. The player must get trains from point A to point B by setting signals to green and clearing the tracks for the train. The player also controls trackswitches. The goal is to ensure trains run on time and don’t collide with each other. The game features two levels. The first level consists of two stations, and the second level has three. The aim of the game is to achieve the highest score, which is displayed on a scoreboard with Daily, Weekly, and Lifetime scores. The art style of the game is simple, reflecting the basic screen of a train traffic controller interface, but we wanted to add a bit more detail than usual.
Signaller was originally designed for a museum and for a large screen with a touchscreen. The game is playable on a desktop, but the experience is best on a large touchscreen display (with multi-touch input support). Features such as inactivity timers are included in the regular version as well.
Project Info:
Team members: David van Rijn & Robin van den Dungen
Project Time: Year 4 period 4 (2023)
Engine: Unity
Code Languages: C#
Design Patterns: Singleton & FlyWeight
Tools
While working on Signaller, I mainly focused on developing tools for building the levels. These tools made the level creation process much faster. Although they were simple buttons, they handled a lot in the background for setting up the rail network. You could create and move RailNodes before and after other RailNodes. Later, I added the "Enter Rail Placemode" feature, which allowed you to place a RailNode at the point where you clicked with your mouse. This tool made building levels faster compared to doing it manually. Iterating on level design was also very easy, with a single button to add a signal. The tool could also generate a mesh of the created level, ensuring that the level matched exactly with what the debug view showed. The game also uses vertex painting to indicate the switch state.
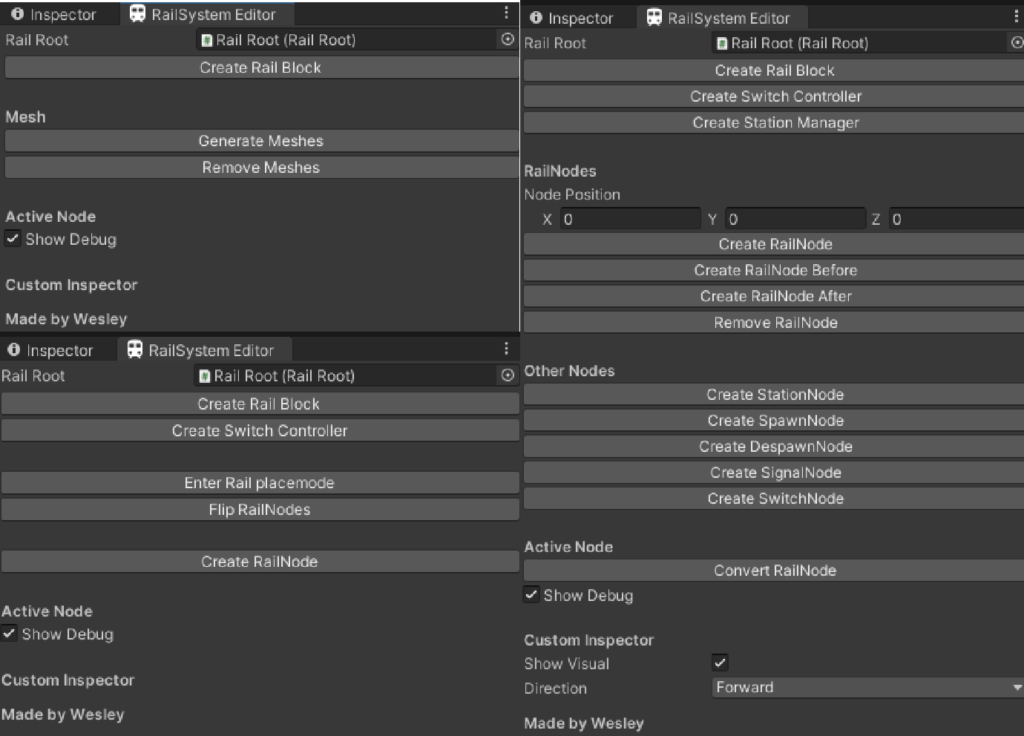
private Transform CreateRailNode(bool isParent, Transform node)
{
Transform nodeTransform = node;
if (!isParent) nodeTransform = node.parent;
RailBlock railBlock = nodeTransform.GetComponent();
RailNode lastRailNode = railBlock.railNodes[railBlock.railNodes.Count - 1];
railBlock.railNodes.Add(CreateNewRailNode(nodeTransform, lastRailNode.transform.localPosition, lastRailNode.transform.localRotation));
GameObject railNode = railBlock.railNodes[railBlock.railNodes.Count - 1].gameObject;
Selection.activeGameObject = railNode;
EditorUtility.SetDirty(railNode);
return railNode.transform;
}
private T CreateOtherNode(string name) where T : RailNode
{
GameObject currentNode = Selection.activeGameObject;
RailBlock railBlock = currentNode.transform.parent.GetComponent();
RailNode currentRailNode = currentNode.GetComponent();
int railNodeIndex = railBlock.railNodes.IndexOf(currentRailNode);
railBlock.railNodes.Remove(currentRailNode);
DestroyImmediate(currentRailNode);
T stationNode = currentNode.AddComponent();
railBlock.railNodes.Insert(railNodeIndex, stationNode);
currentNode.name = $"{name} {currentNode.name.Split(' ')[1].Split("-")[0]}-{railBlock.name.Split(' ')[1]}";
Selection.activeGameObject = stationNode.gameObject;
EditorUtility.SetDirty(stationNode);
return stationNode;
}
With CreateOtherNode, we can create any RailNode that utilizes RailNode, meaning we don’t have to create a function for each individual node.
RailNodes
RailNodes are objects in the scene. They can consist of the following nodes: RailNode, DirectionNode, DespawnNode, ExitNode, SignalNode, SpawningNode, StationNode, and SwitchNode. All nodes are part of a RailBlock, which also manages the mesh of the track. Trains know which RailBlock they are on and which Node they are at. All RailBlocks are contained within the RailRoot, the main object of the RailObjects. Trains can ignore instructions if the direction does not match the traveling direction (DirectionNode).
The SignalNode and SwitchNode were the most complex to implement. Signals can also display orange/yellow, depending on the next signal. However, a switch can cause the next signal to differ from the one that would be "straight ahead." A signal checks the one in front of it but also updates the one behind it. SwitchNodes also send updates to signals to ensure they are always displayed correctly.
private void UpdateSignalsInFront(RailBlock block)
{
int signalIndex = block.railNodes.IndexOf(this);
for (int i = signalIndex + 1; i < block.railNodes.Count; i++)
{
if (block.railNodes[i].GetComponent()) return;
if(block.railNodes[i].TryGetComponent(out ExitNode exitNode))
{
UpdateSignalsInFront(exitNode.transform.parent.GetComponent());
return;
}
if (block.railNodes[i].TryGetComponent(out SignalNode signal))
{
if (signal.nodeDirection == nodeDirection)
{
signal.SwitchUpdate();
return;
}
}
}
}
It goes through the RailNodes and updates the next signal. At an ExitNode, it switches to the RailBlock of the ExitNode.
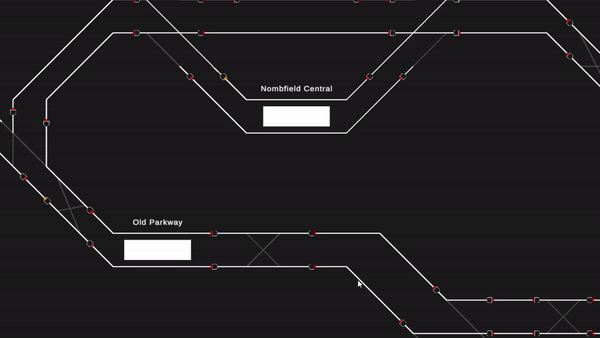
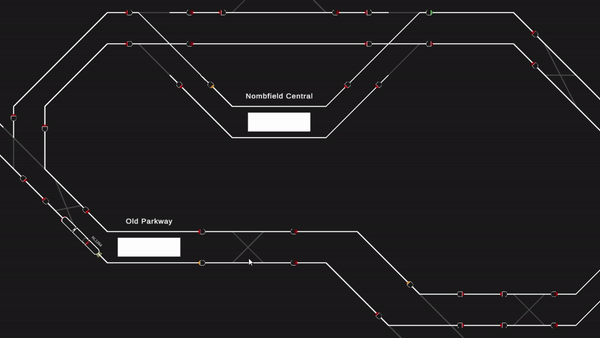